The Art of Clean Code: Principles for Maintainable Software
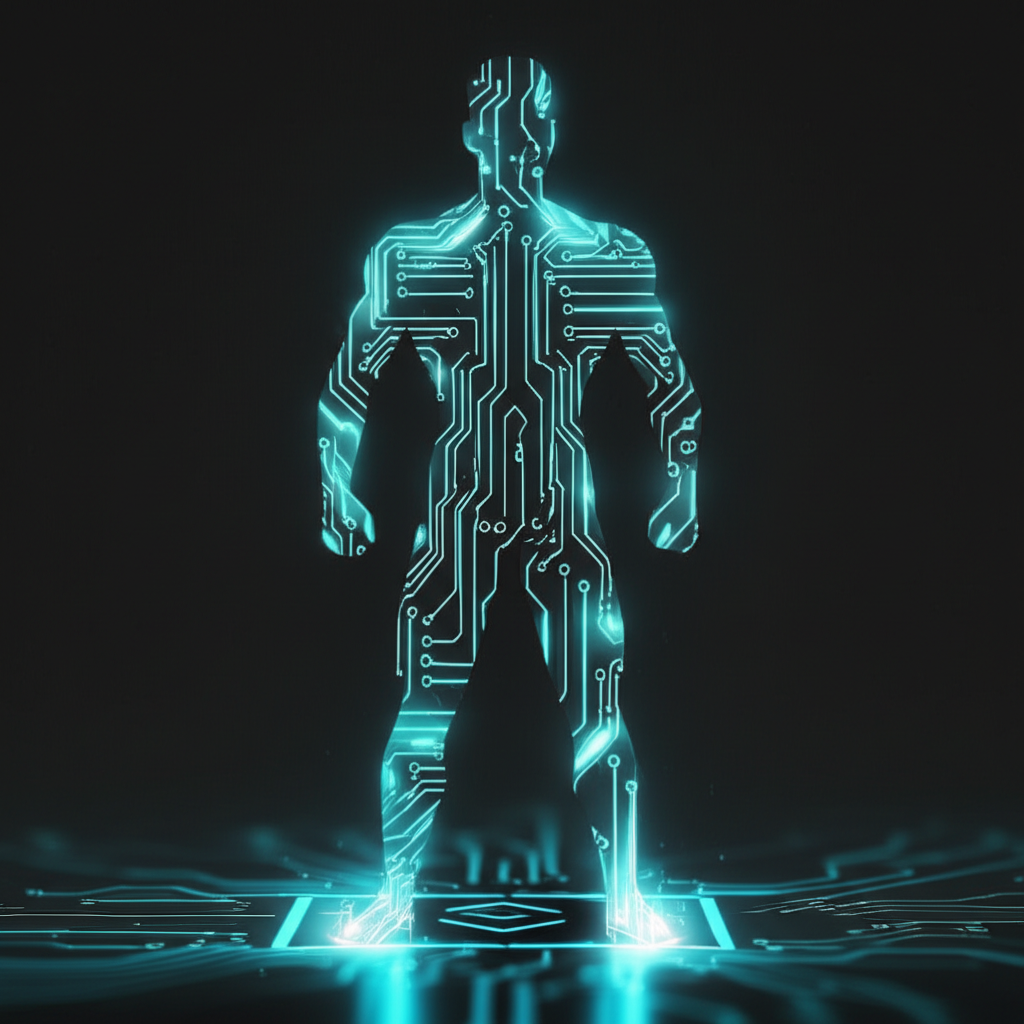
Introduction
As a full-stack developer with experience in PHP/Symfony and React/Next.js, I've come to appreciate that writing code is much more than making something work. Code that merely functions is only half-done. Clean code—code that is readable, maintainable, and adaptable—is the true mark of professional software development.
What Is Clean Code
Clean code is code that is easy to understand, easy to change, and easy to extend. It's code that can be read and understood by other developers (or your future self) without excessive mental effort. It clearly expresses its intent without hiding surprises or requiring extensive documentation. It contains no duplication or redundancy, has minimal dependencies, and is easily testable. Most importantly, it follows consistent patterns and practices that make it predictable and approachable.
Meaningful Names
One of the most fundamental aspects of clean code is using meaningful names for variables, functions, classes, and modules. Names should reveal intent, avoid disinformation, and make meaningful distinctions. A well-named function or variable should tell you what it does or represents without requiring you to look at its implementation. For example, 'getUsersByRole' is much more informative than 'getUsers' or worse, 'get'.
Functions
Functions should do one thing, do it well, and do it only. They should be small, with ideally no more than 20-30 lines of code. They should operate at a single level of abstraction and have descriptive names that clearly communicate their purpose. Function arguments should be limited—fewer arguments make functions easier to understand and test. When a function needs many parameters, consider using parameter objects or builder patterns.
Solid
The SOLID principles provide a foundation for creating maintainable object-oriented code. Single Responsibility Principle (SRP) states that a class should have only one reason to change. Open/Closed Principle (OCP) suggests that software entities should be open for extension but closed for modification. Liskov Substitution Principle (LSP) requires that subtypes must be substitutable for their base types. Interface Segregation Principle (ISP) states that clients should not be forced to depend on interfaces they do not use. Dependency Inversion Principle (DIP) suggests that high-level modules should not depend on low-level modules; both should depend on abstractions.
Refactoring
Clean code often emerges through refactoring—the process of restructuring existing code without changing its external behavior. Common refactoring techniques include extracting methods or classes, renaming for clarity, replacing conditional logic with polymorphism, and removing duplication. Test-driven development (TDD) provides a safety net for refactoring, allowing you to make changes with confidence that you haven't broken existing functionality.
Testing
Clean code is testable code. Writing tests forces you to think about your code's design and dependencies. It also provides documentation through examples and a safety net for future changes. Unit tests should be fast, independent, repeatable, self-validating, and timely (FIRST). Integration tests ensure that components work together correctly, while end-to-end tests validate the system as a whole.
Conclusion
Clean code is not just about aesthetics or personal preference—it's about professionalism and respect for your colleagues and your future self. As developers, we spend far more time reading code than writing it, so investing in code readability pays significant dividends. By following principles like meaningful naming, small functions, SOLID design, and consistent formatting, we can create code that is not only functional but also maintainable and adaptable to changing requirements.